SSO Integration Documentation
View on GitHub1. Overview
The ITC SSO service provides a secure, centralized authentication system for IITB projects. It enables users to log in once and access multiple applications without re-entering credentials.
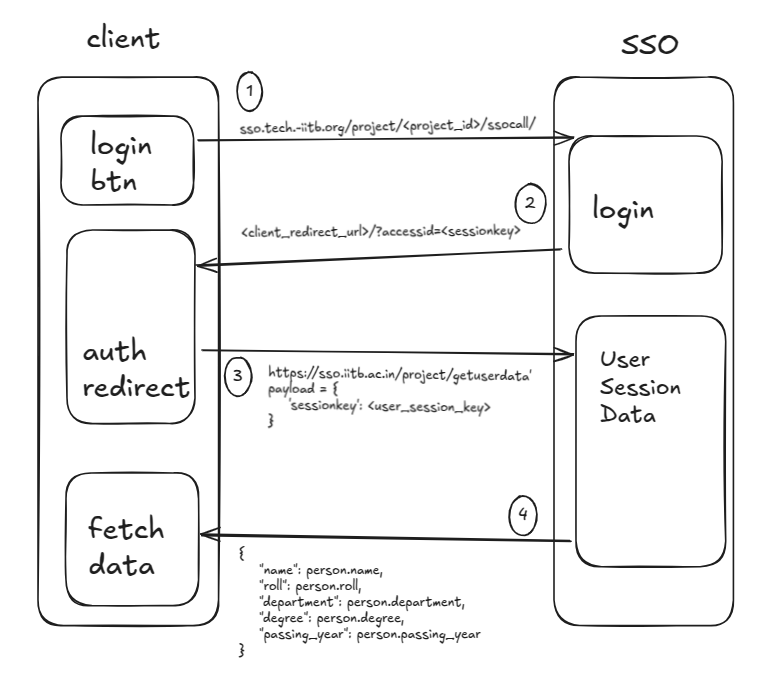
SSO Authentication and Data Flow Diagram
2. Authentication Flow
-
Initial SSO Request:
- Client redirects to:
sso.tech-iitb.org/project/<project_id>/ssocall/
- User is taken to the SSO login page if not already authenticated
- Client redirects to:
-
Authentication Response:
- After successful login, user is redirected to:
<client_redirect_url>?accessid=<session_key>
- The session key is unique for each authentication session
- After successful login, user is redirected to:
-
User Data Retrieval:
- Client makes POST request to:
https://sso.iitb.ac.in/project/getuserdata
- Request body must include the session key
- Client makes POST request to:
-
Data Response:
- SSO returns user profile data in JSON format
- Includes: name, roll number, department, degree, and passing year
3. Integration Steps
- Register your project in the SSO dashboard
- Configure your redirect URL
- Implement the authentication flow
- Handle the user data response
4. Code Examples
Python Example
import requests
# Step 1: Redirect user to SSO
sso_url = f'https://sso.tech-iitb.org/project/{project_id}/ssocall/'
# Redirect user to sso_url
# Step 2: Handle the redirect with session key
# Your redirect endpoint will receive: ?accessid=
# Step 3: Retrieve user data
def get_user_data(session_key):
response = requests.post(
'https://sso.iitb.ac.in/project/getuserdata',
json={'sessionkey': session_key}
)
return response.json()
# Response format:
# {
# "name": "User Name",
# "roll": "123456789",
# "department": "Computer Science",
# "degree": "BTech",
# "passing_year": "2024"
# }
JavaScript Example
// Step 1: Redirect to SSO
function redirectToSSO(projectId) {
window.location.href = `https://sso.tech-iitb.org/project/${projectId}/ssocall/`;
}
// Step 2: Handle the redirect and get user data
async function handleSSORedirect() {
const urlParams = new URLSearchParams(window.location.search);
const sessionKey = urlParams.get('accessid');
if (sessionKey) {
const userData = await getUserData(sessionKey);
console.log('User Data:', userData);
}
}
// Step 3: Fetch user data
async function getUserData(sessionKey) {
const response = await fetch('https://sso.iitb.ac.in/project/getuserdata', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ sessionkey: sessionKey })
});
return response.json();
}
5. Error Handling
Common Error Responses
- 400 Bad Request: Missing or invalid session key
- 401 Unauthorized: Invalid or expired session
- 403 Forbidden: Project not authorized
- 404 Not Found: Invalid endpoint or project ID
6. File Storage
All media files (including profile pictures) are stored securely using MinIO object storage. Files are organized in buckets:
- Media Files: Stored in the media bucket with appropriate access controls
- Static Files: Served from a dedicated static bucket